State management refers to how an application’s state/data is stored, updated, and synced across components over time. State typically includes things like user data, API responses, form fields, UI toggles, etc.
State management becomes crucial as web apps grow more complex with dynamic UIs that update based on user interactions and backend data changes.
Table of Contents:
What is State Management for?
Using state management in JavaScript applications helps to:
- Manage global state in a predictable and traceable way.
- Reduce bugs caused by the shared state.
- Simplify scaling applications.
- Optimize performance by minimizing unnecessary re-renders and updates.
- Synchronize application data.
State Management Approaches
Traditional state management:
Managing the state of the application directly in the code. The only method that does not require additional infrastructure. Therefore, it is the easiest to implement but the hardest in scaling and further management.
Container state management:
Using a container to manage the state of the application. A container is a separate process that manages the application’s state, and the application accesses the state through a defined interface. This approach adds complexity to the system as a whole but is more scalable.
Service state management:
A service manages the state of the application. A service is a separate process or module that manages a specific aspect of the application’s state. This method has similar characteristics to containers.
Event-driven state management:
Managing the state of the application through events. An event represents a change in the application’s state, updated in response to each event. This method is the most flexible but is extremely difficult to understand and debug.
State management libraries:
This method involves using a library to manage the state of the application. These libraries help simplify the state management process by providing a set of tools and patterns. Easier scaling and load balancing made this method the most popular among all mentioned above.
Some popular state management libraries include Redux, MobX, Vuex, React Context, and Angular Services. Let’s dive into three most popular ones.
Redux
Redux is a state management library for JavaScript applications. It helps developers manage global state by providing a single source of truth for the application’s state.
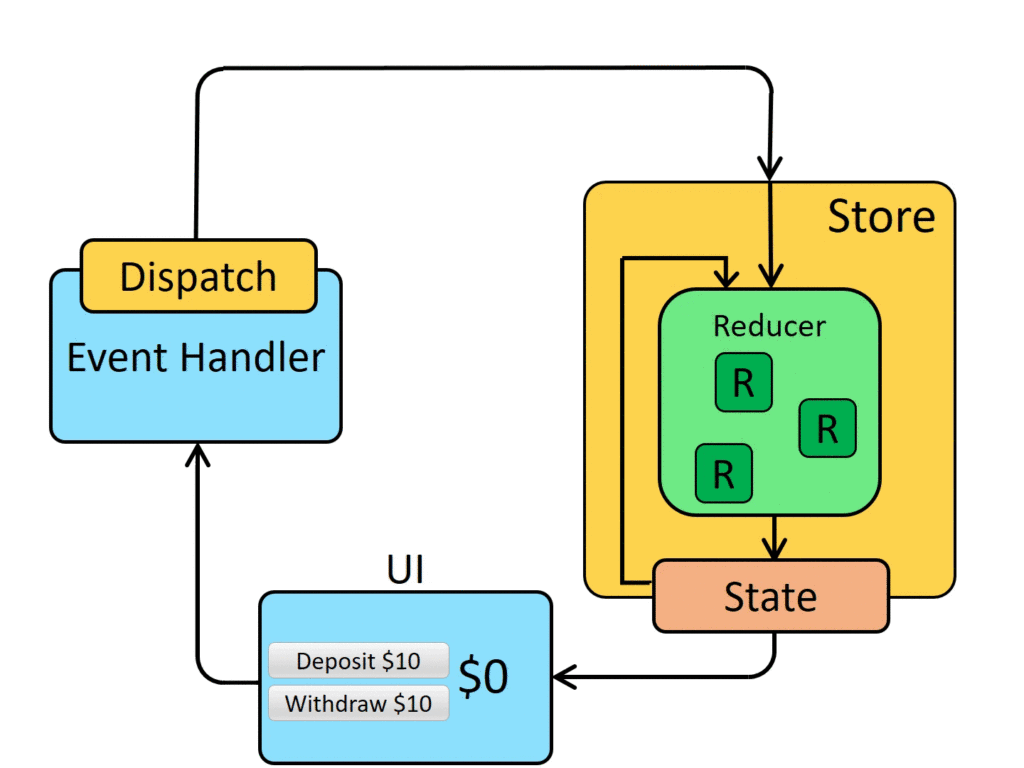
Redux is inspired by the Unix philosophy of “Do One Thing and Do It Well” and aims to provide a simple and reliable way to manage state.
Core Principles of Redux:
- Single Source of Truth:
Redux maintains a single centralized store that holds the entire state of the application to ensure that there is a clear, consistent source of data for the entire app, and debug, test, and scale applications easily. - The State is Read-Only:
The state in Redux is immutable. To make state changes, you must dispatch actions that describe what happened. Reducers then handle these actions and produce a new state, preserving the previous state. - Changes are Made with Pure Functions:
Redux uses pure functions to update the state. Pure functions take the current state and an action and return a new state for a better understanding of how the state is updated and debugging.
Pros and Cons of Redux
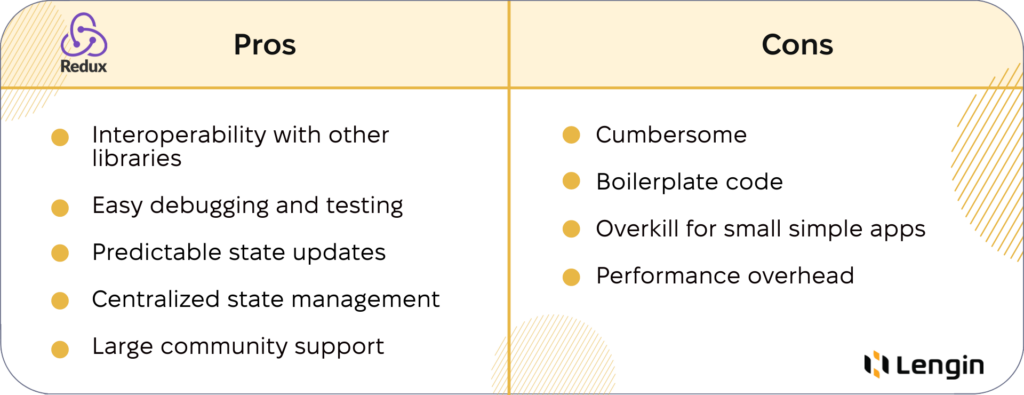
Redux can introduce performance overhead, as the state updates are performed through a centralized store, and the state must be re-rendered whenever it changes. Also, Redux can require a significant amount of boilerplate code, especially when setting up the store, actions, and reducers, making the app more cumbersome and complex.
Business Case: META
META includes many applications with billions of users. Since engineers from META are talented enough to invent React, they created Redux for state management. Therefore, it will be the perfect example of a business case.
We use Redux to manage state on instagram.com, so at a high level, the way we implemented this was to store a subset of our Redux store on the client in an indexedDB table and then rehydrate the store when the page first loads.
Glenn Conner
It works in the following way. The application’s state is lost when the user closes their browser or clears their cache. Consequently, when the user opens the application again, the client-side code (JavaScript) detects that the Redux store is empty or missing and initiates the rehydration process.
The client-side code uses IndexedDB to retrieve the saved state of the Redux store from the user’s browser. The retrieved state is then used to rehydrate the Redux store on the client side, effectively restoring the application’s state to its previous state.
The rehydrated Redux store is then used to render the application’s UI and handle user interactions. Mission complete!
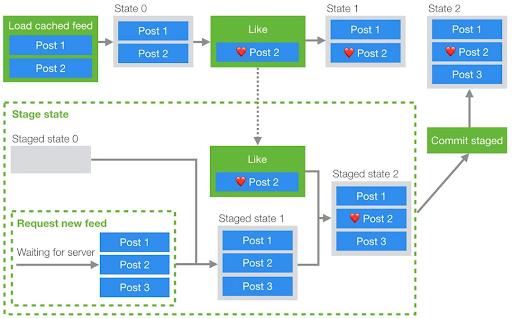
Also, Redux powers state coordination across the complex web and mobile versions and is involved in the following ways too:
Building out dynamic profiles, image/video galleries, direct messaging, and shop/sponsored content plugins involve lots of coordinated states. Redux’s single source of truth approach would be invaluable for an experience with many interactive pieces.
Advanced AR/VR worlds (Oculus VR) have state demands approach beyond traditional apps. Redux would help manage synchronized global entity states for user avatars, environments, and multi-user interactions across all VR/AR devices.
META is a real giant in its industry, but specially-built-for-this Redux handles all the aspects perfectly.
Mobx
MobX is primarily used to simplify state management in dynamic user interfaces. It leverages JavaScript’s runtime to automatically track data dependencies and updates in a declarative way.
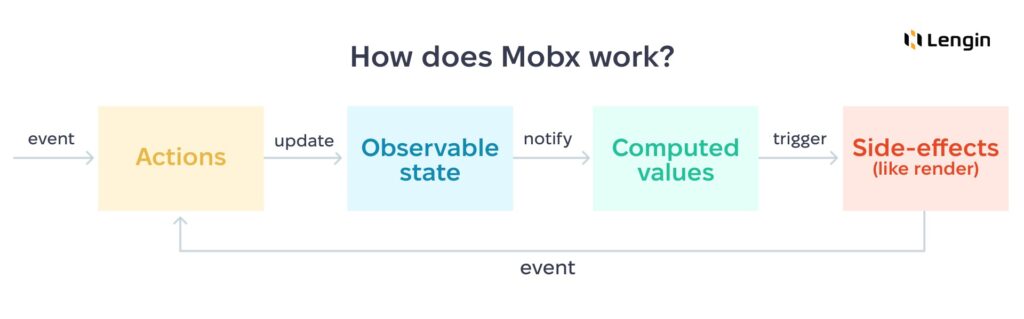
Fundamental Concepts of Mobx:
- Observables:
These are the pieces of data (objects, arrays, and values) that are observed for changes. When observables change, MobX automatically triggers updates in any components that depend on them. - Actions:
Actions are functions that modify observables. They are the only way to change the state of an observable in MobX. Actions ensure that changes are tracked correctly and reactively. - Reactions:
Reactions are functions that automatically run according to the changes in observables. They allow you to define what should happen in response to changes in your application’s state.
Pros and Cons of Mobx
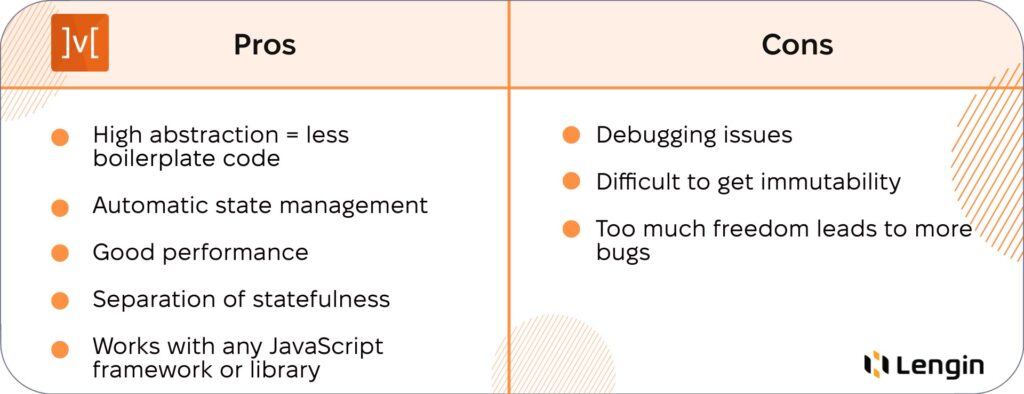
MobX has a high level of abstraction, which significantly reduces boilerplate code compared to Redux, making the app more lightweight. Also, MobX allows for separation of statefulness of the app for component rendering, making the code more legible and easier to maintain.
Business Cases: Udemy and Canva
Udemy uses MobX to manage the state of its course catalog and student progress. They have a large and complex application with many different components that need to interact with each other, and MobX helps them manage the state of these components in a centralized and predictable way.
In particular, Udemy uses MobX to manage the following aspects of their application:
- Course catalog:
Storing and managing the state of their course catalog includes information about each course, such as its title, description, and price. - Student progress:
Tracking students’ course progress, including their completion status and any awards or certificates they have earned. - Search and filtering:
Managing the state of their search and filtering features allows users to find courses based on specific criteria such as topic, price, and duration.
Canva is a graphic design platform that uses MobX to manage the state of its design editor. The editor allows users to create and customize graphics, and MobX helps Canva manage the state of the design in real time.
In particular, Canva uses MobX to manage the following aspects of their design editor:
- Design elements:
Storing and managing the state of design elements such as text, images, and shapes. This includes their position, size, and styling. - Design history:
Tracking the history of changes made to the design allows users to easily undo and redo changes. - Collaboration:
Managing the state of collaborative editing, allowing multiple users to work on the same design simultaneously
In these examples, Mobx’s reactive nature enables scale-building of reactive, dynamic UIs. It streamlines state coordination between disparate features, teams, and codebases – key factors for Udemy and Canva scope platforms that serve many users simultaneously.
Vuex
Vuex is a state management pattern and library specifically designed for Vue.js applications.
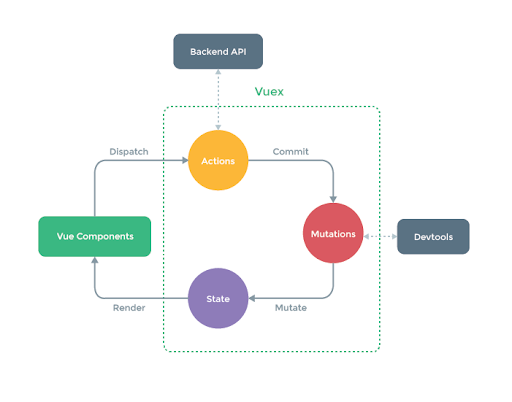
Core Principles of Vuex:
- Single Source of Truth.
All data is stored in one place. - Data is Read-Only.
This means that the only way to change the state is by committing mutations. - Mutations are Synchronous.
- Mutations are functions that modify the state in a Vuex store. They must be synchronous, which means they execute one after another in the order they are called.
Pros and Cons of Vuex
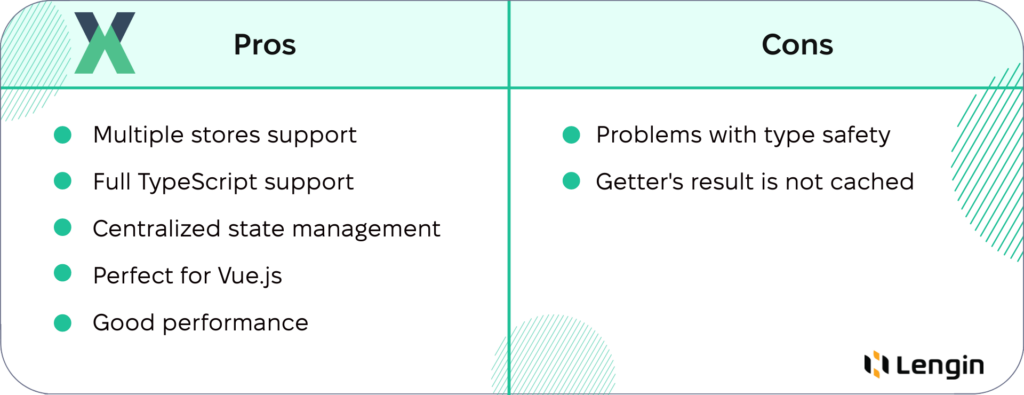
Vuex is the recommended library for state management by the Vue core team. However, Vuex 4 has some problems associated with type safety and, as of Vue 3, the getter’s result is not cached.
Business Cases: UpWork and Deleo Korea
Whether you’re a freelancer looking for gigs on Upwork or browsing the Deleo Korea site, there’s a good chance the amazing user experiences you encounter utilize Vuex for state management behind the scenes. Vuex is perfect for applications built with Vue.js, so obviously, UpWork and Deleo Korea chose it for dealing with their complex state management.
Deleo Korea is a SaaS-based global e-commerce logistics integrated IT platform. Their platform must handle complex logistics data from multiple retailers, products, warehouses, and countries.
Mutations in Vuex are initiated by specific interactions inside the platform that help to keep all the operation statuses up-to-date – whether it is retailer rate or order tracking.
Upwork is one of the largest online freelancing platforms. Their single-page app needs to handle a lot of complex user, project, and account-related data all over the site for both freelancers and clients. Vuex manages the state in a centralized way and allows the building of reusable components.
For example, a freelancer’s profile page may pull details from the store instead of directly from an API, making it easier to share that data and keep it up to date everywhere it’s used.
In both these examples, Vuex streamlines complexity and improves performance by avoiding prop drilling down through multiple levels. A shared store makes complex workflows like user logins, multi-step forms, order tracking, and more simpler. The developer experience and maintainability are improved since there’s a single source of truth for any piece of data.
Both Upwork and Deleo Korea appreciate the structure and organization that Vuex brings to their code, as well as its ability to handle complex data flows and async actions.
General Table of Comparison: Redux vs. Mobx vs. Vuex
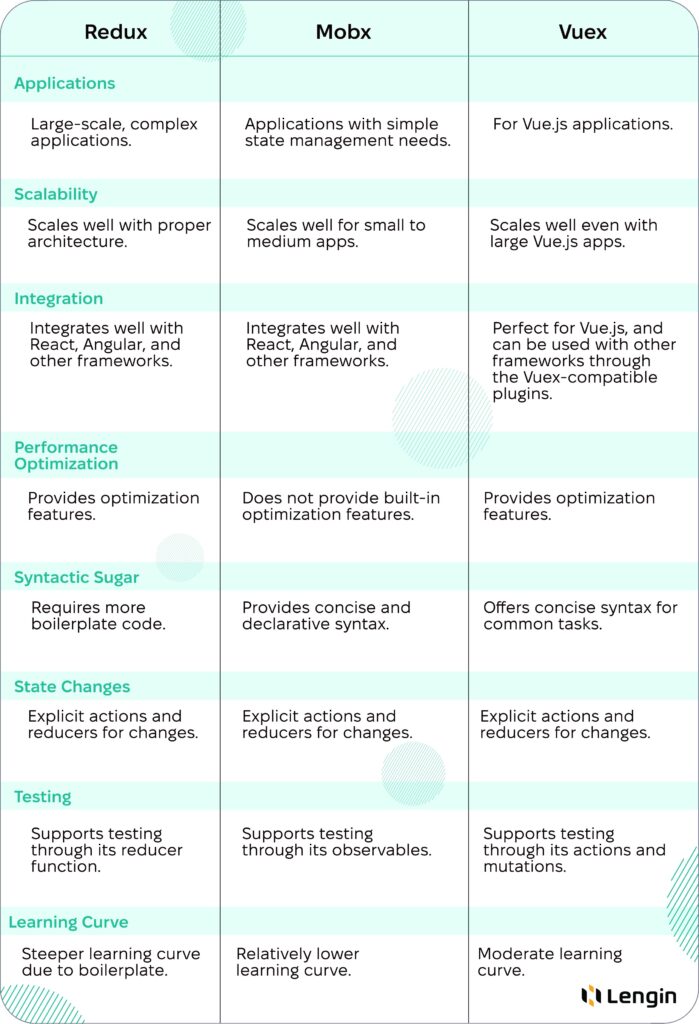
Conclusion
Libraries like Vuex and Redux follow a pattern of centralized, single-source-of-truth state stores using actions or mutations. This declarative structure scales gracefully to large codebases, enabling features like hot reloading, time-travel debugging, and immutability. Their robust ecosystems benefit collaborative teams maintaining complex experiences through many development cycles.
Alternatively, MobX emphasizes reactivity from plain objects without as much ceremony. For some, this provides a gentler framework, particularly during early prototyping. Mobx still handles growing demands via optimized change detection.