React is not a programming language, as many may have thought. It is not a framework, as some may claim. React is an open-source JavaScript library.
The best way to describe how React works is through the quote of outstanding programmer:
“Write programs that do one thing and do it well. Write programs to work together.”
Douglas McIlroy
That one thing for React is building a User Interface – the combination of buttons, panels, and other interactive elements on the dashboard that the user sees first.
Table of Contents:
The contemporary state of React
React has a rapidly expanding supportive community that significantly contributes to further improvements. In 2023 React took a new direction – moving more code blocks from the client to the server side. It can affect in several ways:
- Improved performance.
The client’s device can now focus on rendering the user interface and handling interactions instead of processing extensive data. - Reduced bandwidth usage.
It is possible because of minimizing data transfers between client and server. - Enhanced security.
All sensitive operations are kept on the server. - Code reusability and scalability.
The usage of the same logic across different client applications and platforms is available now.
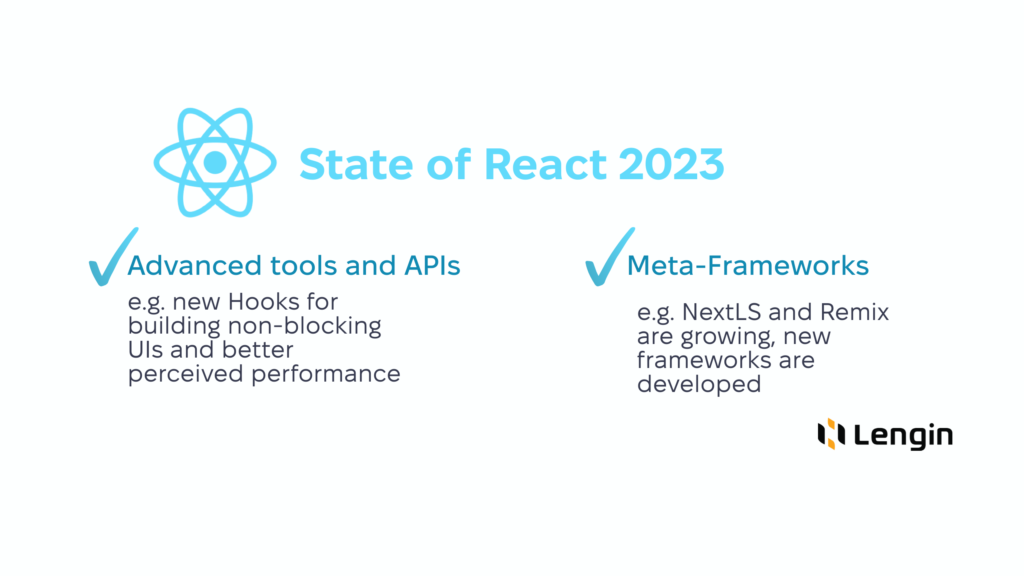
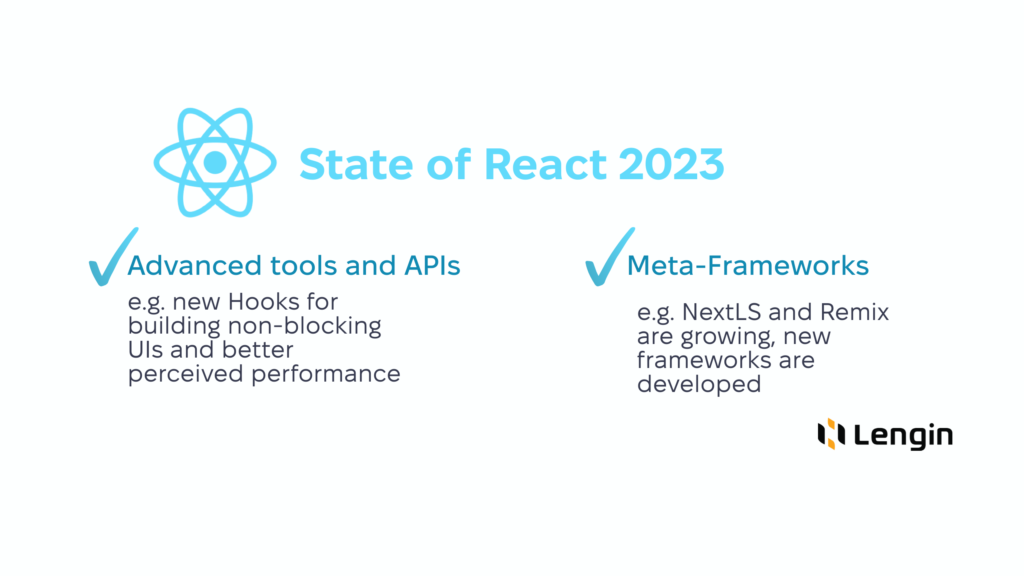
New advanced tools, hooks, and meta-frameworks are only the beginning. So what is React and how does it work?
React Сomponents
Since React’s key fundamental is the creation of reusable components, UI can be quickly built out of these blocks. They are self-contained modules that encapsulate a piece of functionality.
Due to this feature, developers can implement each block several times across not only the same but different applications, modifying them according to the new requirements. After creation, rudimental components can be combined into more functional ones with a specific purpose.
Except for encapsulating and compositional characteristics, the separation of concerns is another one worth attention to. Separation of concerns means that each component focuses on its designated task, and matters are distributed among distinct components.
Breaking down complex tasks into smaller, more focused parts makes understanding, testing, and maintaining code less troublesome. This promotes a collaborative development workflow, enabling different team members to work on separate components without conflicting with each other’s code.
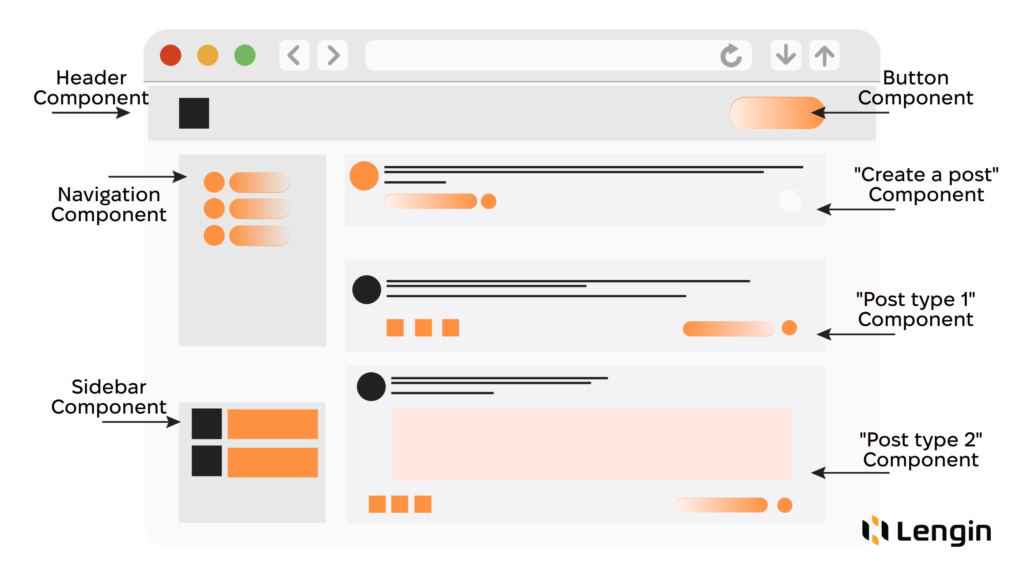
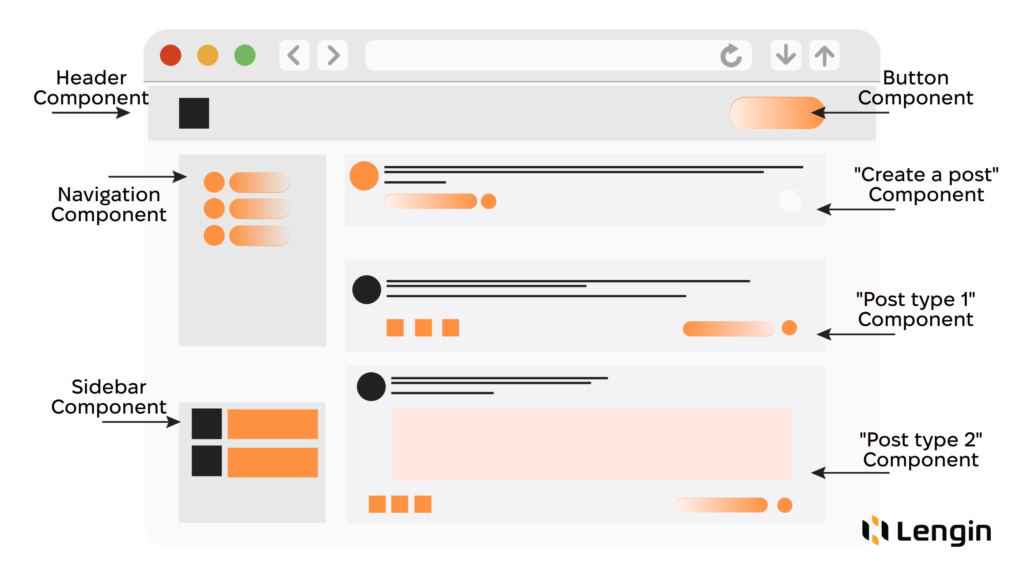
There are two types of components: functional and class. Class components are more cumbersome but used to provide more complex functionality that functional components couldn’t. Everything changed with releasing React Hooks.
React Hooks
React 16.8 introduces React Hooks, which enable functional components to behave like class components. They are functions that allow you to “hook into” React state and lifecycle functionality within functional components. React Hooks became a technological breakthrough that bright the set of improvements to the React applications:
- Facilitate development.
The simplification of logic re-usage, state handling, and side effects performing makes the development faster and the code more adaptive. - Separation of concerns.
Assigning a unique task for each functional component makes the code more concise and modular. - Benefits during writing tests.
Testing of the components does not entail any risks due to the possibility of testing the behavior of each Hook in isolation without adverse impact on the whole system. - Improve performance.
It is possible because of eliminating the need for creating new instances of class components for each render.
The best approach would be to choose the type of components depending on the requirements of your specific application. Although functional components are generally recommended for simplicity and ease of use, there may be situations in which class components may be necessary to achieve more complex functions.
After building the application’s skeleton block by block and imbuing it with functionality, it’s time to tap into Virtual DOM and make all these come to life.
Virtual DOM
The DOM, which stands for Document Object Model, is a programming interface that provides a structured representation of HTML and XML documents.
React creates a Virtual DOM – an optimized simulated replica of the actual DOM to avoid unnecessary actions. React re-renders the component and builds a brand-new virtual DOM tree. After that, it calculates the minimal changes required to update the actual DOM based on the updated virtual DOM tree. This process is called “reconciliation.”
As a result, React can efficiently modify only the areas with changes without re-rendering the entire system. This reduces the number of manipulations required to update the actual DOM, resulting in a lower UI updating cost.
To facilitate the creation and management of the Virtual DOM, React introduces JSX, a syntax extension that blends JavaScript and XML.
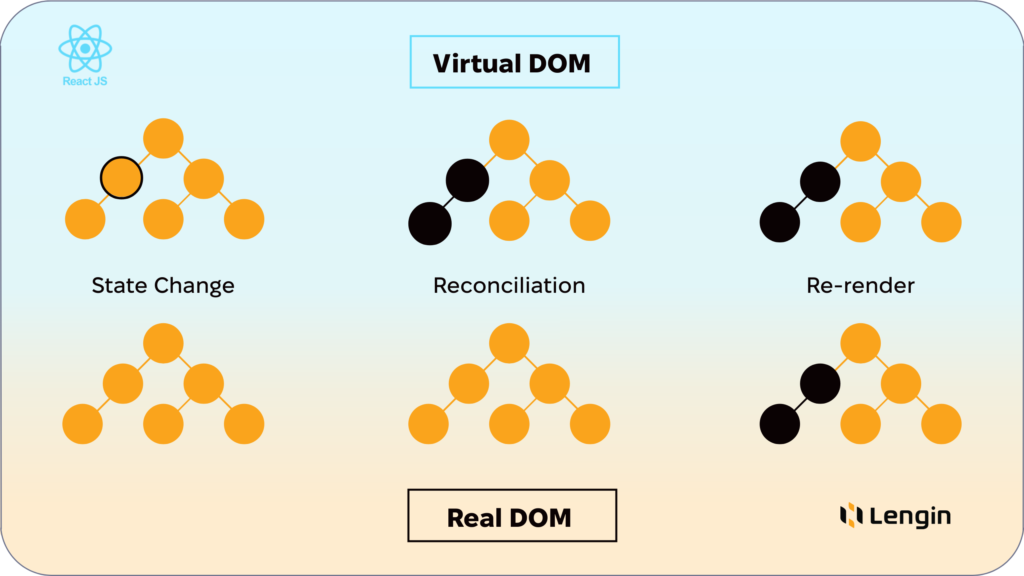
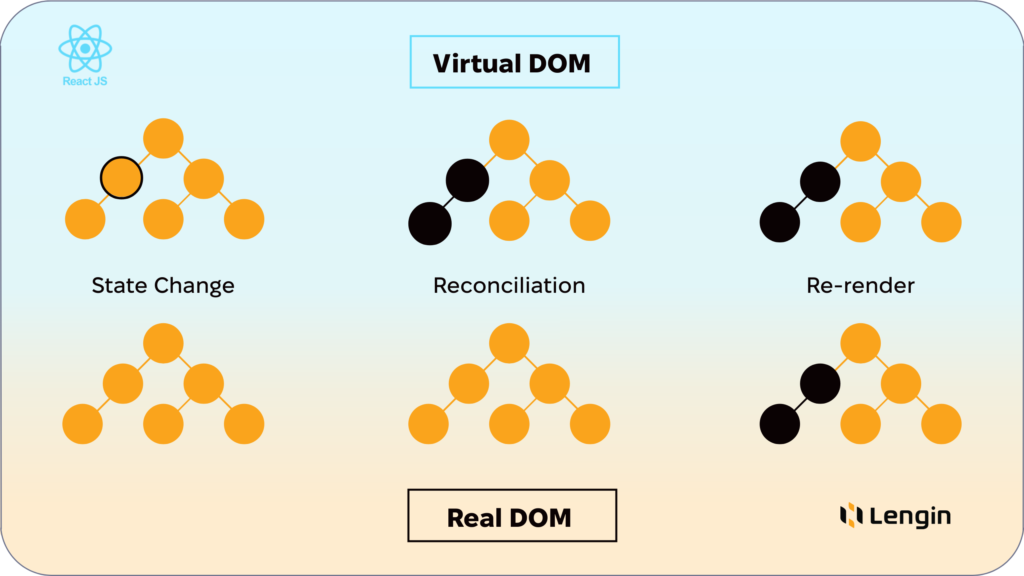
JavaScript XML
Instead of writing traditional HTML tags, React uses JSX, which stands for JavaScript XML. They have a lot of similarities with some slight syntax differences and also some added functionality. JSX makes writing and managing complex UI logic in your applications more manageable and makes the code more legible, especially for designers and non-engineers.
Using JSX for your application’s UI can help you bring the product to market faster, maintain and modify the code more efficiently, improve collaboration with designers, build more robust and reusable components, and ensure quality through testing. These factors can improve business outcomes like revenue, productivity, and customer satisfaction.
React Ecosystem
React exists in the substantial functional ecosystem of web development tools, frameworks, and other libraries that offers many benefits in terms of productivity, code quality, and scalability. There are the most widely used of them:
React Native for Mobile Development
React Native enables building cross-platform mobile applications with a native look and feel that work seamlessly on both iOS and Android devices, using a single JavaScript codebase mixed with native platform components. The React Native framework is equipped with a robust collection of tools and libraries, simplifying the process of building intricate user interfaces, managing data, and generating native animations.
Material UI as One of the Best ReactJS UI Frameworks
Despite many other practical design libraries in the React ecosystem, Material UI is the most known for successfully implementing Google’s Material Design guidelines. Virtually, it includes a flexible and customizable set of components for buttons, cards, forms, icons, navigation, and more that can be easily styled to fit the needs of a particular project. It also implies features like server-side rendering, support for themes, and accessibility features out of the box.
Next.js Framework
Next.js framework provides many features that facilitate the building of fast and scalable web applications:
- Automatic code splitting.
Optimized application performance due to ensuring that only the necessary code is loaded. - Simplifies image handling.
This feature allows efficient and optimized image processing. - Built-in support for CSS modules and Sass.
Management of styles is more efficient now because of modular and reusable styling components. - The server-side rendering.
Code execution on the server side enhances performance, reduces server load, and results in better performance and SEO.
Redux – a Predictable State Container
It is one of the most used tools with JavaScript applications and React. Redux facilitates the analysis of how data flows through an application. By providing a clear separation between the state and the UI, Redux helps make applications more maintainable and easier to test. It also enables time-travel debugging, stepping through the history of an application’s state changes to see how it evolved over time.
GraphQL vs. Traditional REST APIs
GraphQL is an open-source query language and runtime for APIs designed as a more efficient and flexible alternative to REST APIs. Using GraphQL, clients can specify exactly what data they want from the server, eliminating over-fetching or under-fetching issues. It also simplifies API evolution, allowing clients to adapt to changes without requiring server modifications.
Error Boundaries as an Instrument of Error Handling
React provides a mechanism called “Error Boundaries” that allows developers to handle errors that occur in rendering components in a more structured way. Error Boundaries are particular components that wrap up other components and can catch errors that occur during the rendering process.
By using Error Boundaries in this way, developers can create a more robust and resilient application by providing fallback UIs or error messages that help users understand what went wrong and how to recover from errors. This can help improve the overall user experience and reduce frustration when things go wrong.
The flexibility of approach and variety of available assets made React a beneficial solution for building UI for many business applications.
Business Case: META
Various companies, with no exaggerations, giants in their industries, use React and its ecosystem constituents for scalable solutions, exceptional user experience, and efficient data management.
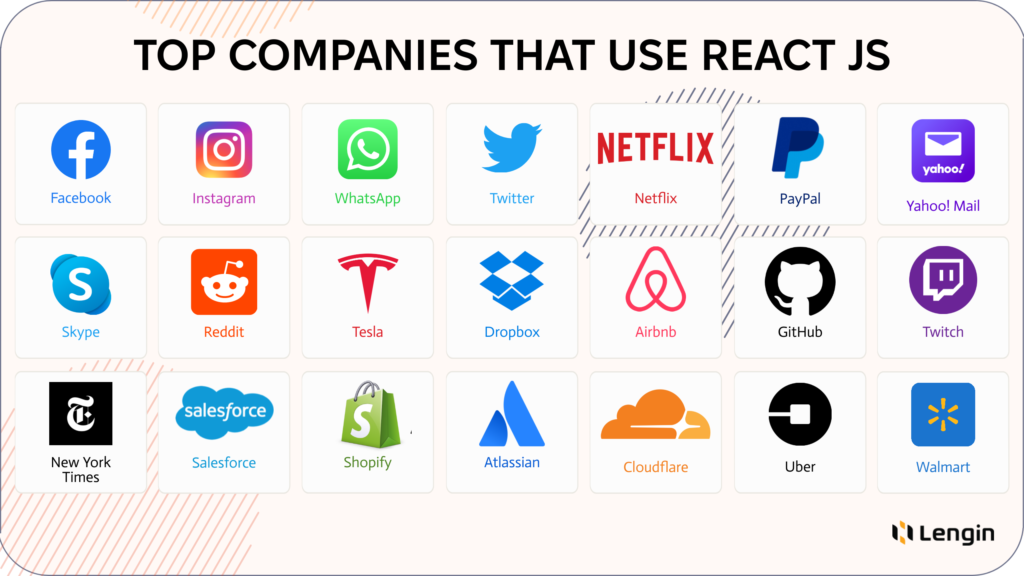
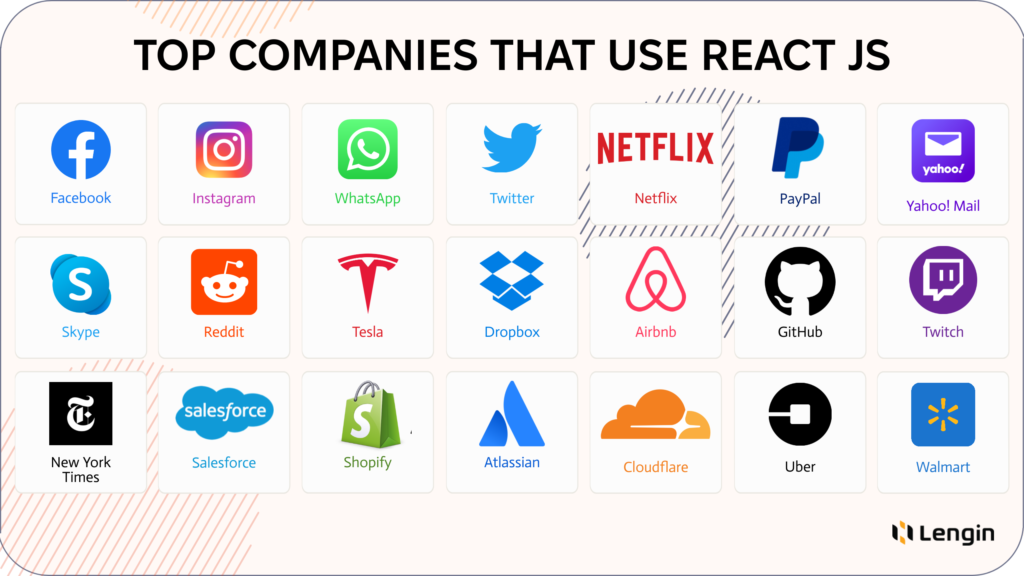
Since React was developed by Facebook to fulfill their need, Meta would be a perfect example of successfully implementing the best React practices in real life.
React Native for Mobile Applications
Instagram, Facebook, Messenger, and WhatsApp can efficiently function on both iOS and Android devices with perfectly adapted to the screen size interactive interface.
Web versions of Facebook and Instagram
React’s declarative syntax and component model make it easier to manage even such a complex user interface with a large number of posts and interactions of a platform like Instagram without adverse effects on user experience and resource consumption.
Oculus VR
Virtual reality is a new trend. Oculus VR platform utilizes React VR and creates immersive Metaverse experiences using React’s component-based approach.
Redux
This state management library is primarily engaged with Ads Manager. Redux helps manage and synchronize the vast amount of data associated with advertising campaigns across various components.
React Router
React Router enables seamless navigation between different pages and components, providing a smooth user experience.
Testing Frameworks
Meta employs various testing frameworks from the React ecosystem, such as Jest and React Testing Library, to ensure the quality and reliability of their applications.
Those are examples of tools and components that can be used, but the React ecosystem can fulfill all the needs of any application thanks to the wide variety of possible sets of tools and features.
Final Thought
React has become one of the most popular and widely used technologies in web development and continues to push the boundaries of what’s possible. Since the library constantly evolves, improving performance and tooling with every release, React will likely remain a key technology for building modern, high-performance web applications. React’s innovative approach and a large ecosystem of tools and libraries make it a terrific solution for creating modern UI components and applications.
Contact us, and let’s build engaging and interactive web applications with React!